#sudo apt install python3 python3-pip
#sudo pip3 install selenium
#pip install webdriver-manager
# Variables
YoutubeUrl="https://www.youtube.com/watch?v=7nT5YawZt-s"
TotalWaitSeconds=10
import time
import selenium
from datetime import datetime
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from selenium.webdriver.common.action_chains import ActionChains
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.common.by import By
from selenium.webdriver.support import expected_conditions as EC
# This section spawns Chrome with a Youtube URL, then maximizes its player
def playYoutube(url,waitTime):
options = webdriver.ChromeOptions()
options.add_experimental_option("useAutomationExtension", False)
options.add_experimental_option("excludeSwitches",["enable-automation"])
options.add_experimental_option("detach", True)
driver = webdriver.Chrome(options)
wait = WebDriverWait(driver, waitTime/2)
driver.get(url)
ActionChains(driver).move_to_element(wait.until(EC.visibility_of_element_located((By.CSS_SELECTOR, "div.ytp-chrome-controls")))).perform()
maximize_button_visible=wait.until(EC.visibility_of_element_located((By.CSS_SELECTOR, "button.ytp-fullscreen-button.ytp-button")))
driver.maximize_window()
time.sleep(waitTime/2)
maximize_button_visible.click()
#wait.until(EC.visibility_of_element_located((By.CSS_SELECTOR, "button.ytp-fullscreen-button.ytp-button"))).click()
#time.sleep(30)
playYoutube(YoutubeUrl,TotalWaitSeconds)
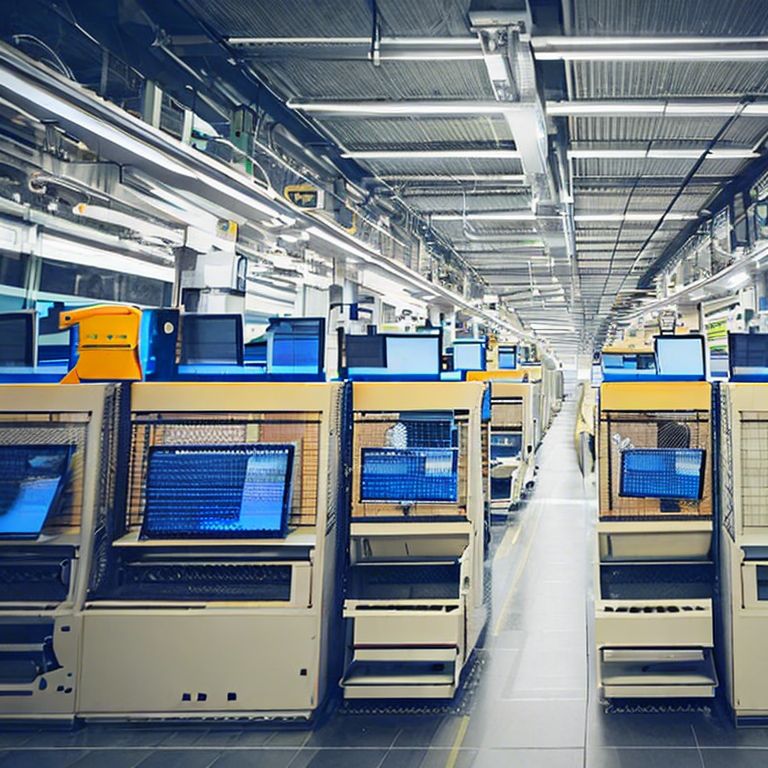
June 12, 2024June 12, 2024
0 Comments