Prerequisites:
OS: base image Centos 7
Create network bridge from command line:
# Network: x.x.x.128/26 Range: x.x.x.128-190 broadcast: x.x.x.191
docker network create -d macvlan \
–subnet=192.168.30.0/24 \
–gateway=192.168.30.254 \
–ip-range=192.168.30.128/26 \
-o parent=enp3s0f0 \
qa-vlan
# show result
docker network ls
Install Portainer:
# Create persistent volume
docker volume create portainer_data
# check created volumes
ls /var/lib/docker/volumes/
# Generate certs (optional and assuming no HAProxy)
openssl genrsa -out portainer.key 2048
openssl ecparam -genkey -name secp384r1 -out portainer.key
openssl req -new -x509 -sha256 -key portainer.key -out portainer.crt -days 3650
# Input these values
#Country Name (2 letter code) [XX]:US
#State or Province Name (full name):California
#Locality Name (eg, city) [Default City]:Long Beach
#Organization Name (eg, company) [Default Company Ltd]:kimconnect
#Organizational Unit Name (eg, section):Cloud
#Common Name (eg, your name or your server’s hostname)]:server01.kimconnect.com
#Email Address:[email protected]
# move files into /local-certs
mkdir /var/lib/docker/volumes/portainer_data/certs
mv ~/portainer.crt /var/lib/docker/volumes/portainer_data/certs
mv ~/portainer.key /var/lib/docker/volumes/portainer_data/certs
# SELinux configuration – Enable IP forwarding
sysctl net.ipv4.conf.all.forwarding=1
sudo iptables -P FORWARD ACCEPT
# Run portainer with priviledged mode, SSL, persistent volume and company logo
docker run -d –privileged -p 9000:9000 –name portainer –restart always -v /var/lib/docker/volumes/portainer_data/certs:/certs -v /var/run/docker.sock:/var/run/docker.sock -v portainer_data:/portainer_data portainer/portainer –ssl –sslcert /certs/portainer.crt –sslkey /certs/portainer.key –logo “http://kimconnect.com/uploads/logo.png”
# Configure firewall to open port 9000
# First discover the active zone of current machine
firewall-cmd –get-active-zones
# Open port 9000 to the public zone and reload firewalld
firewall-cmd –zone=public –add-port=9000/tcp –permanent
firewall-cmd –reload
Access Portainer via browser at the host’s IP
Manage Endpoints:
LDAP Integration:
Assuming that LDAP is already available in the environment. Portainer can be configured to use LDAP authentication as follows:
{Settings} > {Authentication} > {LDAP}
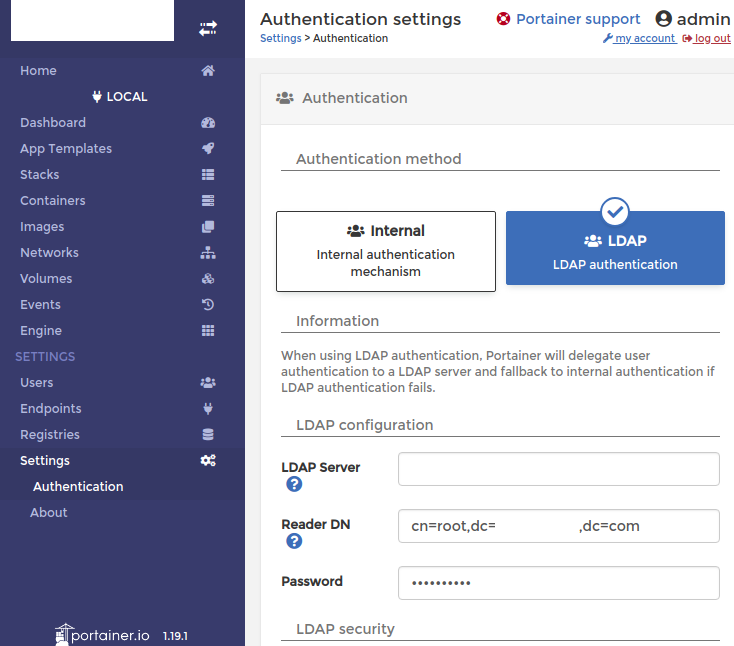
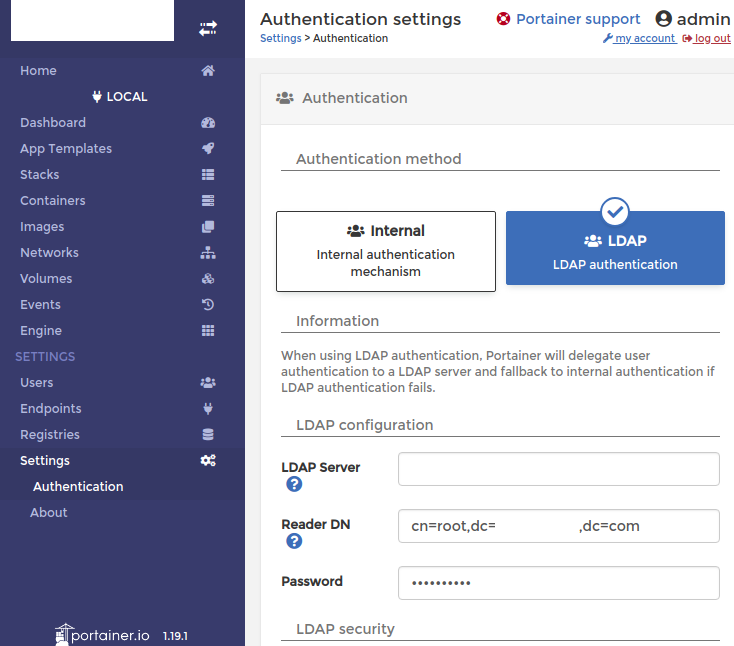
LDAP Server: LDAP-SERVER:PORTNUMBER
Reader DN: cn=root,dc=kimconnect,dc=com
Password: standard
Use TLS: true
Skip verification: true
base DN: dc=kimconnect,dc=com
Username attribute = uid
Filter: (objectClass=*)
Group Base DN: ou=people,dc=kimconnect,dc=com
Group Membership: memberOf
Group Filter: (objectClass=*)
Click {Test Connection} > {Save settings} > use a different browser to test login with an LDAP account to verify access
Dockerfile (Sample)
-
vim ~/Dockerfile
FROM ubuntu:16.04
RUN apt-get update && apt-get install -y curl vim ntp ntpdate cvs rsync git wget openssh-server tar iputils-ping tzdata
ENV TZ=America/Los_Angeles
RUN ln -snf /usr/share/zoneinfo/$TZ /etc/localtime && echo $TZ > /etc/timezone
RUN mkdir /var/run/sshd
RUN echo ‘root:[insert-password-here]’ | chpasswd
RUN sed -i ‘s/PermitRootLogin prohibit-password/PermitRootLogin yes/’ /etc/ssh/sshd_config
# SSH login fix
RUN sed ‘s@session\s*required\s*pam_loginuid.so@session optional pam_loginuid.so@g’ -i /etc/pam.d/sshd
ENV NOTVISIBLE “in users profile”
RUN echo “export VISIBLE=now” >> /etc/profile
EXPOSE 22
CMD [“/usr/sbin/sshd”, “-D”]
-
docker build -t ubuntu16_baseline .
Working with Repos
# Change into webadmin as that account has been given sudo access as required to build images
su – webadmin
# Check to see if ssh key is available
cat .ssh/id_rsa.pub
# If not, generate ssh key
ssh-keygen -t rsa
# view the ssh key
cat .ssh/id_rsa.pub
# Copy the output (key string) and paste it into
# Gitlab profile keys:
# Prepare projects folder and clone the git repo
mkdir /home/webadmin/projects
cd /home/webadmin/projects
git init
git clone [email protected]:docker/app1.git
cd app1/
# build the image
sudo docker build -t app1 .
# After image named “app1” is built
# Access Portainer UI to create a container with that image
Working with Docker via CLI
Creating a Baseline Image of Ubuntu 16
# Download Ubuntu 16.04 LTS
[root@docker]# docker pull ubuntu:16.04
# Check Image_ID of Ubuntu
[root@docker]# docker images
REPOSITORY TAG IMAGE ID CREATED SIZE
ubuntu 16.04 b9e15a5d1e1a 29 hours ago 115MB
portainer/portainer latest 6827bc26a94d 5 weeks ago 58.5MB
alpine latest 11cd0b38bc3c 2 months ago 4.41MB
# Clone the Ubuntu image by initiate its Image_ID to obtain a new instance_ID
[root@docker]# docker run -i -t b9e15a5d1e1a /bin/bash
root@77fc4f0f4910:/# exit
exit
[root@docker ~]# docker ps -a
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
77fc4f0f4910 b9e15a5d1e1a “/bin/bash” 2 minutes ago Exited (0) About a minute ago dreamy_fermi
172eae6e386a portainer/portainer “/portainer –ssl –…” 33 minutes ago Up 33 minutes 0.0.0.0:9000->9000/tcp portainer
de82f3306700 alpine:latest “/bin/sh” 2 hours ago Up 31 minutes alpine-test
# Modify cloned Ubuntu container and merge changes to the downloaded Ubuntu 16.04 image
docker start 77fc4f0f4910
docker attach 77fc4f0f4910
apt-get update
apt-get upgrade
apt-get install -y curl vim ntp ntpdate cvs rsync git wget openssh-server tar
ntpdate -u -s 0.north-america.pool.ntp.org 1.north-america.pool.ntp.org 2.north-america.pool.ntp.org 3.north-america.pool.ntp.org
# Set root password
root@77fc4f0f4910:/# passwd
Enter new UNIX password:
Retype new UNIX password:
passwd: password updated successfully
# enable ssh-root-login
sed -i ‘s/prohibit-password/yes/’ /etc/ssh/sshd_config
service ssh reload
# Setup OpenSSH to autostart (this settting doesn’t persist)
systemctl enable ssh
exit
docker commit 77fc4f0f4910 ubuntu:16.04
# Check to verify that the modified Ubuntu image has been committed
[root@docker ~]# docker image ls
REPOSITORY TAG IMAGE ID CREATED SIZE
ubuntu 16.04 0a7e4f1e1fdc 19 minutes ago 304MB
ubuntu <none> b9e15a5d1e1a 29 hours ago 115MB
portainer/portainer latest 6827bc26a94d 5 weeks ago 58.5MB
alpine latest 11cd0b38bc3c 2 months ago 4.41MB
Frequently Used Commands
# Check running containers
docker container ls -a
# How to stop an running VM
docker stop container_name
# Remove a container
docker container rm container_id
# Connect portainer to qa-vlan
docker network connect qa-vlan container_name
# Run a test container based on Alpine Linux (very small)
docker run -itd \
–network qa-vlan \
–name alpine-test \
alpine:latest
# Inspect alpine-test and look for “Networking” node to copy its MAC address
docker container inspect alpine-test
# Discover what the container’s interfaces are showing and check its route
docker exec alpine-test ip addr show eth0
docker exec alpine-test ip route
Working with Portainer GUI
Home > click on an Endpoint > {Containers} > {Add container} > input these variables:
Create container:
Name = remote_automation
Image = ubuntu16_baseline:latest
Always pull the image = true
Publish all exposed ports = true
Enable access control = false
Command:
Console = Interactive & TTY
Volumes:
volume = remote_automation (writeable)
Network:
network = qa-vlan
Hostname = remote-automation
Domain Name = kimconnect.com
IPv4 Address = 192.168.30.101
Restart Policy:
Restart policy = always
Set Memory & CPU limits:
Finally, click {Create container} > wait a few seconds to be redirected back to the Containers List
Old Notes:
# Install docker
yum install -y docker
systemctl enable docker
systemctl start docker
# Run docker
# docker volume create portainer_data
docker run –privileged –restart always –name=portainer -d -p 9000:9000 -v /var/run/docker.sock:/var/run/docker.sock -v portainer_data:/data portainer/portainer
# Check running containers
docker ps -a
# Hide container
docker run -d –label owner=acme portainer
# Change container name
docker rename CONTAINER NEW_NAME
#### Delete all Containers and Images ####
#### Warning: this is irreversible ####
#!/bin/bash
# Delete all containers
docker rm $(docker ps -a -q)
# Delete all images
docker rmi $(docker images -q)
# Create persistent volume
# Remove all unused networks
docker system prune -f
# Remove all unused volumes
docker system prune –volumes -f
# Remove all stopped containers
docker container prune -f
# Remove all unused images
docker image prune -f
# Stop and remove all containers
docker container stop $(docker container ls -aq)
docker container rm $(docker container ls -aq)
docker volume create portainer_data
cd /var/lib/docker/volumes/
mkdir portainer_data
# mkdir cert && cd cert
# Run portainer
docker run -d –privileged -p 9000:9000 –name=portainer –restart always -v portainer_data:/portainer_data portainer/portainer -v /var/run/docker.sock:/var/run/docker.sock
docker run -d –privileged -p 9000:9000 –name=portainer –restart always -v portainer_data:/portainer_data portainer/portainer -v /var/run/docker.sock:/var/run/docker.sock -v /var/lib/docker/volumes/portainer_data/certs:/certs
# Set the Admin username and password:
# Troubleshooting firewall – use as needed
iptables -A INPUT -i docker0 -j ACCEPT
iptables -I INPUT 4 -p tcp -m state –state NEW -m tcp –dport 9000 -j ACCEPT
iptables -A INPUT -p tcp -m tcp –dport 9000 -j ACCEPT
# Enable IP forwarding
sysctl net.ipv4.conf.all.forwarding=1
iptables -P FORWARD ACCEPT
firewall-cmd –permanent –zone=public –add-port=9000/tcp
# Test SSL connection
openssl s_client -connect kimconnect.com:443 -prexit | less
# Stop/remove all running containers
docker stop $(docker ps -aq)
docker rm $(docker ps -aq)
# Remove all images
docker rmi $(docker images -q)
# Check logs
docker logs –tail 50 –follow –timestamps CONTAINERNAME
#################################################### OLD INFO #################################################
#### Install Docker ####
# Cleanup yum
yum clean all
# List repos
yum repolist
# enable Extras
vim /etc/yum.repos.d/CentOS_Base.repo
# Add enabled=1 into the Extras repos section
yum -y update
# Install prerequisites
sudo yum install -y yum-utils device-mapper-persistent-data lvm2
# Add Docker repo
yum-config-manager –add-repo https://download.docker.com/linux/centos/docker-ce.repo
# Install Docker-CE
yum -y install docker-ce docker-ce-cli containerd.io
# Start Docker
systemctl start docker
# “Mount Drive” driver
curl -fsSL https://raw.githubusercontent.com/CWSpear/local-persist/master/scripts/install.sh | sudo bash
#### General SSL cert and key ####
openssl x509 -outform der -in /etc/letsencrypt/live/kimconnect.com/fullchain.pem
-out /etc/letsencrypt/live/kimconnect.com/kimconnect.crt
openssl pkey -in /etc/letsencrypt/live/kimconnect.com/privkey.pem -out /etc/letsencrypt/live/kimconnect.com/kimconnect.key
#### Install Portainer ####
docker volume create portainer_data
ls /var/lib/docker/volumes
mkdir /var/lib/docker/volumes/portainer_data/certs
docker run -d –privileged -p 9000:9000 –name portainer –restart always -v “/var/run/docker.sock:/var/run/docker.sock” -v ~/local-certs:/certs -v portainer_data:/data portainer/portainer:latest –ssl –sslcert /etc/letsencrypt/live/kimconnect.com/kimconnect.crt –sslkey /etc/letsencrypt/live/kimconnect.com/kimconnect.key
docker run -d –privileged -p 9000:9000 –name portainer –restart always -v “/var/run/docker.sock:/var/run/docker.sock” -v portainer_data:/data portainer/portainer:latest -l owner=acme
# With Logo
$ docker run -d -p 9000:9000 -v /var/run/docker.sock:/var/run/docker.sock portainer/portainer –logo “https://www.docker.com/sites/all/themes/docker/assets/images/brand-full.svg”
# mypassword is plaintext here
$ echo -n mypassword > /etc/letsencrypt/live/kimconnect.com/portainer_password
$ docker run -d -p 9000:9000 -v /var/run/docker.sock:/var/run/docker.sock -v /tmp/portainer_password:/tmp/portainer_password portainer/portainer –admin-password-file /tmp/portainer_password
# Firewall stuff
firewall-cmd –permanent –zone=public –add-port=9000/tcp
firewall-cmd –permanent –zone=public –add-port=80/tcp
firewall-cmd –permanent –zone=public –add-port=443/tcp
firewall-cmd –reload
firewall-cmd –permanent –zone=trusted –change-interface=docker0
firewall-cmd –permanent –zone=trusted –add-port=8000/tcp
firewall-cmd –reload
# Remove container by name
docker container ls
docker stop {instance_ID}
docker rm /portainer
# Resolve maxconnections issue
–ulimit nofile=65536:65536
# OR run at command
ulimit -u unlimited
### Optional Section ###
# optional: add a user into the Docker group
usermod -aG docker kimconnect
# optional: remove and cleanup docker
yum remove docker-ce
rm -rf /var/lib/docker
# Remove older versions of Docker
yum remove docker docker-client docker-client-latest docker-common docker-latest docker-latest-logrotate docker-logrotate docker-engine